1) Introduction
In the Creating and instancing .NET assemblies by using VBScript driver [1] article, you can see how to create a .NET assembly on Microsoft Visual C# and load it into E3 via VBScript Driver. This feature can be used, for example, to communicate to web services.
This article will explain how to create an assembly that communicates to a web service. To simulate the example’s communication, we will use the Elipse WebService Driver, which creates a web service in the computer executing it.
2) Adding a reference to the web service
First, you must create a project on Microsoft Visual C#, setting it according to the previously mentioned article [1]. It is really important that you do not forget to enable the Com-Visible option in the assembly’s options.
In this example, the project will be called WebServiceClient, and the class will be called Client.
To create the reference, you must publish the web service with access permissions. Thus, you have to create an E3 project with a configured and activated Elipse WebService Driver.
In the Microsoft Visual C# 2008, the communication to a web service can be performed by in two ways:
- Service Reference: this options creates a client class to communicate to services based on Windows Communication Foundation (WCF) [3], which can be web services or not. The .NET Framework 3.0 or higher is required.
- Web Reference: creates a client class to communicate to web services described on on Web Services Description Language (WSDL) [4]. It is based on .NET Framework 1.1 or 2.0.
Since the web service created by the Elipse WebService Driver is described on WSDL, we will create a Web Reference. To do so, in the created project, click the Project – Add Service Reference menu. On the next window, click the Next… button and then click the Add Web Reference button (Figure 1).

After inserting the web service’s URL created by the Driver (which you can get on the Elipse WebService Driver’s settings) and clicking the Go button, the retrieved services are displayed (in this case, SendData). To add a reference to the project, just click the Add Reference button (Figure 2).
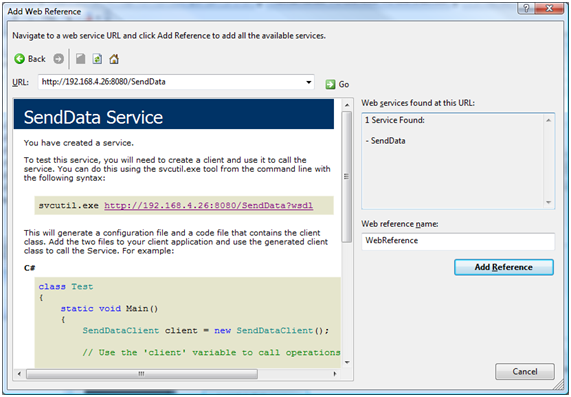
Thus, a class enabling communication with the service and calling the available methods is created. In the Client class’ code below, a WebReference.SendData variable and two methods are created: the SendData method sends a data to the first position of the objects array expected by the web service’s SetParams functionmethod, and the Dispose method makes sure that the resources will be freereleased.
namespace WebServiceClient
{
public sealed class Client : IDisposable
{
private WebReference.SendData service;
public Client()
{
service = new WebServiceClient.WebReference.SendData();
}
public void SendData(object o)
{
object[] obj = new object[1] { o };
service.SetParams(obj);
}
public void Dispose()
{
service.Dispose();
service = null;
}
}
}
3) Accessing the web service via VBScript Driver
After having compiled the assembly, you can load it via scripts, according to the article [1]. However, besides the DLL file, an XML file and another DLL file are also generated, and they must be in the same directory: the XML file sets the web service’s settings (WebServiceClient.dll.config), and the DLL file is used in the XLM’s serialization (WebServiceClient.XmlSerializers.dll).
Then, you must add a new Driver object to the E3 project to load the VBScript Driver. To send data to the web service, you must also have to create a tag with writing permissions, and the Item parameter must be set to be acknowledged by the script (the name “Tag” was used), according to the Figure 3. The script below creates a WebServiceClient.Client object when the dDriver is activated, and sends the tag’s value by using the SendData method when it is written:
Dim obj
Sub OnStart()
Set obj = CreateDotNetObject(“C:\WebServiceClient.dll”, “WebServiceClient.Client”)
End Sub
Sub OnWrite_Tag(r, v)
obj.SendData(v.Value)
End Sub
Sub OnStop()
Set obj = Nothing
End Sub

In the example, when writing on the Tag1, the value must be displayed on the Element1 of the block created in the Elipse WebService Driver (Figure 4). As you can see, we have written Test, on it, which was read via web service and displayed on the Element1.
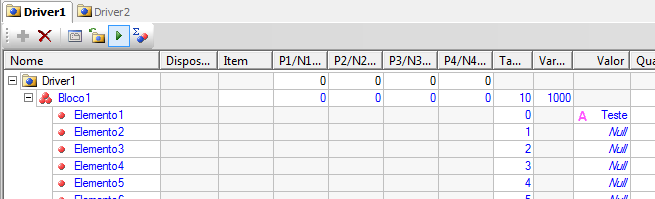
Figure 4. Value read by the Driver via web service
4) Conclusion
By loading .NET assemblies and instancing the configured data types, it is possible to make the scripts’ development with complex tasks easier. For example, you can use the .NET Framework to communicate to several types of web services.
For more information about the settings of configuring references to services oin the Microsoft Visual C#, check the MSDN Library [5] [6].
5) References
[1] Creating and instancing .NET assemblies by using VBScript driver
[2] Enviando dados ao E3 através de web services .NET (in Portuguese)
[3] Windows Communication Foundation (WCF)
[4] Web Services Description Language (WSDL)
[5] How to: Add a Reference to a Web Service
[6] How to: Add, Update, or Remove a Service Reference