GENERAL NOTES:
- This article applies to Elipse E3, versions 4.5 or higher.
- For further details on E3DataAccess library, we recommend reading the following articles: Connecting a VBA application to an E3Server using the E3DataAccess library and BUG-0013588: Incompatible types when calling E3DataAccess’ GetValue/SetValue methods.
Introduction
This article uses JavaScript and VBScript languages to demonstrate how to use E3DataAccess library. In the examples, the scripts were used on an HTML page, and the client is Internet Explorer version 11.
Languages such as JavaScript offer no support to passing parameters as reference, and that is why JavaScript was used alongside VBScript to use a few methods of E3DataAccess library.
Internet Explorer version 11 or higher offer unlimited support to VBScript. For further information, access Microsoft’s documentation. To allow VBScript to be used, the following excerpt was added to the page:
Since JavaScript does not support passing parameters as reference, the E3Item class was created in VBScript to use ReadValue and WriteValue methods. This class has two functions: Read(ItemName), which receives as parameter the server tag’s name and then uses ReadValue function to read this tag; and Write(ItemName, vTimestamp, vQuality, vValue), which receives as parameter the name of the server tag where the other parameters Timestamp, Quality and Value will be saved, and writes this data in the server via WriteValue function.
Class E3Item Public Value Public Timestamp Public Quality Public Function Read(ItemName) Read = E3DataAccess.ReadValue(ItemName, Timestamp, Quality, Value) End Function Public Function Write(ItemName, vTimestamp, vQuality, vValue) Write = E3DataAccess.WriteValue(ItemName, vTimestamp, vQuality, vValue) If Write Then Timestamp = vTimestamp Quality = vQuality Value = vValue End If End Function End Class
E3DataAccess Library Methods
1. ReadValue method
To read data, we’ve created Read() function in JavaScript:
function Read() { E3DataAccess.Server = document.E3Config.editServer.value; var msg; //Cria instancia var da = createNewE3Item(); if (da.Read(document.E3Config.editTag.value)) { document.E3Config.editValue.value = da.Value; msg = document.E3Config.editTag.value + ": " + da.Timestamp + ", " + da.Quality + ", " + da.Value; } else { msg = "Error in reading value from server: " + E3DataAccess.Server + " for item: " + document.E3Config.editTag.value; } WriteText(msg); } WriteText(msg); }
This function creates an instance of E3Item class, sends the name of the tag set up by the user in the HTML file to the class’ Read function, and then connects to E3Server, reading Value, TimeStamp and Quality parameters via E3DataAccess’ ReadValue method. Having done that, the values are ready to be used in JavaScript and become available to the user.
2. WriteValue method
Similarly to the read procedure, to write data you will use Write function, created in JavaScript:
function Write() { E3DataAccess.Server = document.E3Config.editServer.value; var msg; //Cria instancia var da = createNewE3Item(); //Formata data para salvar var date = new Date(); var month = date.getMonth()+ 1; var dateString = month + "/" + date.getDate() + "/" + date.getFullYear() + " " + date.getHours() + ":" + date.getMinutes() + ":" + date.getSeconds(); if (da.Write(document.E3Config.editTag.value, dateString, 192,document.E3Config.editValue.value)) { msg = "Writing to server: '" + E3DataAccess.Server + "' for item: '" + document.E3Config.editTag.value + "' succeeded"; } else { msg = "Error in writing value to server: '" + E3DataAccess.Server + "' for item: '" + document.E3Config.editTag.value + "' = " + document.E3Config.editValue.value; } WriteText(msg); }
This function also creates an instance of E3Item class, passes the informed parameters by the user to to the class’ Write function, and uses E3DataAccess’ WriteValue method to write in the tag, thus passing all parameters to E3Server.
3. ExecuteQuery method
You can also create queries via E3DataAccess’ ExecuteQuery method. To do so, we’ve created GetQueryValues() function in JavaScript:
function GetQueryValues() { E3DataAccess.Server = document.E3Config.editServer.value; var da = createNewE3Query(); if (document.E3Config.editQuery.value != "") { da.ProcessQuery(document.E3Config.editQuery.value, "dvTableRended"); document.getElementById("dvTableRended").innerHTML = da.Content; } else { document.getElementById("dvTableRended").innerHTML = Date() + " - Error empty query."; } }
This function creates an instance of E3Query class, created in VBScript according to the example above, using ProcessQuery function. Through this other function, it will use the library’s ExecuteQuery method to query in E3Server.
Class E3Query Public Content Public Function ProcessQuery(queryName, tablePlacement) If queryName <> "" Then Dim vNames, vValues, arrResult If E3DataAccess.ExecuteQuery(queryName, vNames, vValues, arrResult) Then Call RenderTable(arrResult, tablePlacement) Else Document.getElementById(tablePlacement).innerHTML = Now & " - Error in the query result." End If Else Document.getElementById(tablePlacement).innerHTML = Now & " - Error empty query." End If End Function
4. RegisterCallBack and UnregisterCallBack methods
To use these methods, we’ve created RegisterCallback() function in JavaScript. Unlike the functions previously mentioned here, this function doesn’t need to call any methods developed in VBScript, using the library’s RegisterCallBack method directly via JavaScript.
function RegisterCallback() { E3DataAccess.Server = document.E3Config.editServer.value; if (document.E3Config.editTag.value != "") { var result; result = E3DataAccess.RegisterCallback(document.E3Config.editTag.value); WriteText("Register tag: " + document.E3Config.editTag.value + " result: " + result); } else { window.alert("Error! There is no tag item for register. Type one before proceed."); }
And like RegisterCallback method, we’ve also created UnregisterCallback function in JavaScript, as seen below.
function UnregisterCallback() { if (document.E3Config.editTag.value != "") if (document.E3Config.editTag.value != "") { var result; result = E3DataAccess.UnregisterCallback(document.E3Config.editTag.value); WriteText("Unregister tag: " + document.E3Config.editTag.value + " result: " + result); } else { window.alert("Error! There is no tag item for unregister. Type one before proceed."); } }
Testing the Application
To test the application, you must first execute the domain with the E3 application, open the HTML page in Internet Explorer (as an Administrator), and grant access to ActiveX.
Having done that, go to the open HTML page and just inform the path of the E3Server tag you wish to read/write, as seen below.
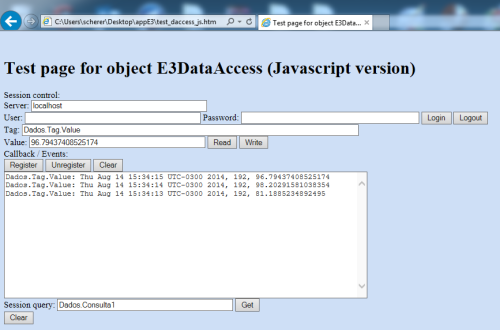
Figure 1. Reading the server tag
To perform a query, just insert (in Session Query) its path in the server, according to the figure below.
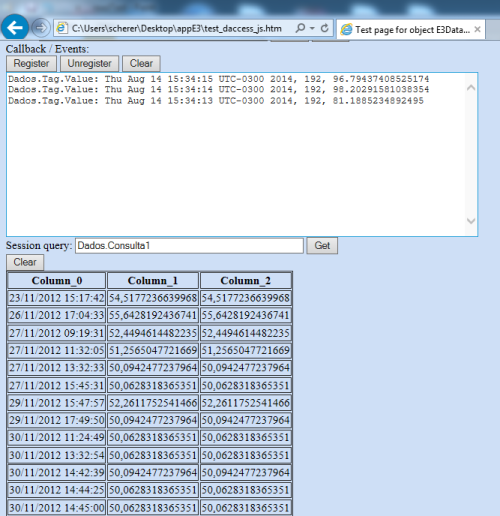
Figure 2. Querying the server
Finally, to use RegisterCallBack and UnregisterCallBack methods, just inser the tag’s path in the server and click Register or Unregister; therefore, whenever there is an event with this tag, it will be displayed on the screen, as seen below.
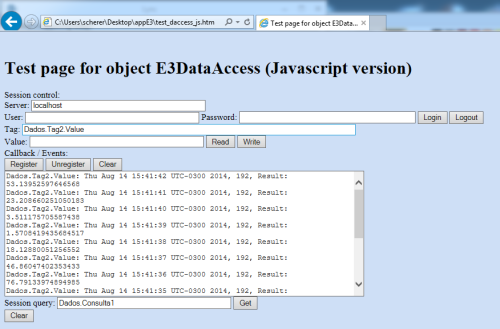
Figure 3. RegisterCallBack in server Tag2. (10)
For further details in the implementation, the E3 demo application and the HTML page used are attached to this article.