Sometimes it is more appropriate to assign more than one value related to a single variable. For this, it is possible to create a variable that contains a series of values, an array-type variable, or a vector.
To declare a variable explicitly, use the Dim command. The declaration of an array uses parentheses containing its dimension.
Example:
Dim A(10)
It is possible to assign data to each of the elements of an array by using an index starting from zero and ending in the declared size (the number of elements of the array is always the number displayed between parentheses plus one).
Example:
A(0) = 256
A(1) = 324
A(2) = 100
…
A(10) = 55
It is also possible to create an array by using the Array(arglist) method from the VBScript. This command returns a variable of the Variant type that contains an array. The values must be separated by commas.
Example:
A = Array(10,20,30)
MsgBox A(0)
MsgBox A(1)
MsgBox A(2)
Split(expression, [delimiter], [count], [compare])
Returns a zero-based one-dimensional array, containing a specific number of substrings.
Expression: Required. String expression containing subsequences and delimiters.
Delimiter: Optional. Any singular character used to identify limits of characters subsequence. If Delimiter is omitted, the space character (” “) is taken as the delimiter.
Count: Optional. Maximum number of substrings that the sequence of input characters should be divided. The default, – 1, indicates that the sequence of input characters should be divided in each occurrence of the Delimiter’s sequence.
Compare: Optional. Numerical value indicating the comparison to use when evaluating substrings.
Example:
MyString = “You can’t judge a book by its cover”
MyArray = Split(MyString, ” “)
‘ MyArray(0) contains “You”
‘ MyArray(1) contains “can’t”
‘ MyArray(2) contains “judge”
‘ MyArray(3) contains “a”
‘ MyArray(4) contains “book”
‘ MyArray(5) contains “by”
‘ MyArray(6) contains “its”
‘ MyArray(7) contains “cover.”
for i=0 to 7
MsgBox MyArray(i)
Next
UBound(arrayname)
Returns the array’s dimension (arrayname).
Example:
Dim MyArray(3)
MsgBox UBound(MyArray)
Displays a message box with the value 3.
Configuring a Driver at Runtime
To change in runtime any property from the I/O driver’s dialog, an array with the parameters to be changed must be created. The most common are:
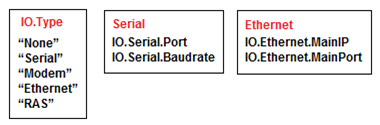
Figure 13
Example:
Dim arr(6)
‘set array’s elements
arr(1) = “IO.Type”
arr(2) = “Serial”
arr(3) = “IO.Serial.Port”
arr(4) = 1
arr(5) = “IO.serial.BaudRate”
arr(6) = 19200
A more elegant way of writing this would be:
Dim arr(3)
arr(1) = Array(“IO.Type”, “Serial”)
arr(2) = Array(“IO.Serial.Port”, 1)
arr(3) = Array(“IO.serial.BaudRate”, 19200)
Exercises:
1. By clicking on a button, create an array with all the days of the week. Keep the array in an internal tag.
2. By clicking on a button, read each position of the array created in the previous exercise, and display them on a message box. To know the array’s size, use the UBound command.
3. Insert a Listbox. In OnStartRunning event, type the following script:
‘Fill ListBox
Clear()
AddItem “Morning,Afternoon,Night”
AddItem “violet,indigo,blue,green,yellow,orange,red”
AddItem “1;2;3;4”
4. Create a button that separates each word and displays them on a message box. Please note that each line contains a different amount of words.
5. Write the required array to set up the driver with the following parameters:
a) Ethernet / 127.0.0.1 / Port 502
b) Serial / COM1 / Baudrate 19200